Download
Download this file as Jupyter notebook: saving_loading.ipynb.
Example: Saving and Loading Objects to Disk
True-Q™ objects can be saved to disk using the trueq.utils.save()
function.
They are saved in the Python pickle
serial binary format and then compressed. After these objects have been saved to disk,
they can be reloaded into Python with the global trueq.utils.load()
function.
The saved file persists beyond the call to load()
and can be
reused.
For CircuitCollection
s, we provide a convenience wrapper that
allows users to save collections by calling the
trueq.CircuitCollection.save()
method.
Independently, many objects also include to_dict
and from_dict
methods, see
for instance to_dict()
and
from_dict()
. The dictionary formats contain only built-in
Python objects.
Note
The file extension .tq
is encouraged, but not necessary.
[2]:
import os
import trueq as tq
Make SRB CircuitCollection on qubit 1, and populate it with results from a simulator.
[3]:
circuits = tq.make_srb(1, [4, 32, 64])
tq.Simulator().add_overrotation(0.05).run(circuits, n_shots=1024)
Save the circuit collection with all metadata, cycles, and results to disk:
[4]:
# use a temporary folder so that this example doesn't make a mess
with tq.utils.temp_folder():
# the extension ".tq" is arbitrary but encouraged
circuits.save("my_circuits.tq")
file_size = os.path.getsize("my_circuits.tq")
print(f"Saved file is {file_size} bytes.")
# load the circuits back to disk
loaded_circuits = tq.load("my_circuits.tq")
Saved file is 11408 bytes.
We have effectively made a deep copy of the original collection:
[5]:
print(loaded_circuits is not circuits and loaded_circuits == circuits)
print(loaded_circuits[0] is not circuits[0] and loaded_circuits[0] == circuits[0])
True
True
In particular, all of the results and circuit contents have been preserved:
[6]:
loaded_circuits.plot.raw()
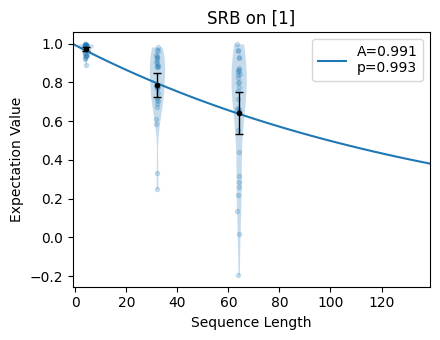
Reducing file size
The filesize can be reduced by choosing to omit all circuit cycles from the saved object, or possibly by saving the fit object instead of the circuits; see the next subsection. This permanently erases this information if the object is also deleted from the active Python session. However, this may be worth it if lots of data is routinely being saved to disk since analysis routines depend only on circuit results and metadata contained in circuit keys, and not on cycles or gates in the circuit.
[7]:
with tq.utils.temp_folder():
# the extension ".tq" is arbitrary but encouraged
circuits.save("my_circuits2.tq", include_cycles=False)
size_ratio = file_size / os.path.getsize("my_circuits2.tq")
print(f"This file is {size_ratio:.2f}x smaller.")
# load the circuits back to disk
loaded_circuits2 = tq.load("my_circuits2.tq")
loaded_circuits2.plot.raw()
This file is 6.56x smaller.
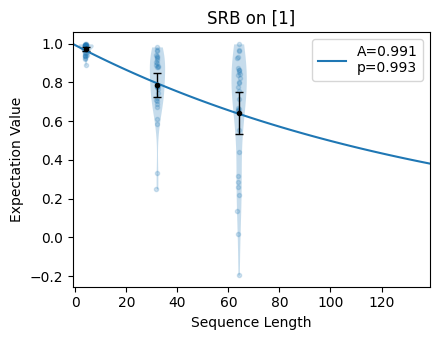
Saving other objects
Many other True-Q™ objects can be stored to disk. Notably, we can store fit results, which may often be an even better method of file size reduction.
[8]:
with tq.utils.temp_folder():
tq.utils.save(circuits.fit(), "my_fit.fit")
size_ratio = file_size / os.path.getsize("my_fit.fit")
print(f"This file is {size_ratio:.2f}x smaller.")
loaded_fit = tq.load("my_fit.fit")
loaded_fit
loaded_fit.plot.raw()
This file is 14.50x smaller.
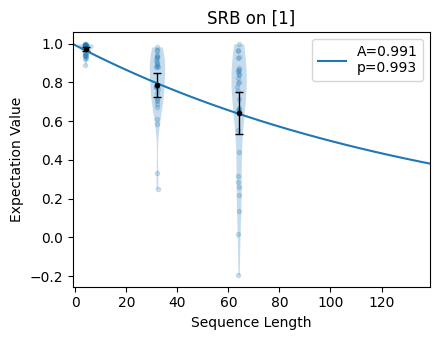
Download
Download this file as Jupyter notebook: saving_loading.ipynb.